Developer : ft-lab (Yutaka Yoshisaka).
06/15/2019 - 03/01/2022.
This script is intended for runtime to build and run.
All the features of the runtime are also available in the Unity Editor.
The demo scenes for runtime is a sample of how to use it.
See also "
Demos for Runtime".
Overview
At runtime, it creates a "Panorama 180-3D" or "Panorama 360-3D" color texture/depth texture from a specified camera.
Provides the ability to get the RenderTexture of a panorama.
It does not have an API for saving file.
Usage : BeginCapture - EndCapture
In this section, the demo scene "Runtime/blocks" will be used as an example.
This is an example of capturing with "BeginCapture()" to "EndCapture()".
Add the script "Panorama180Render" to the camera as a component.
At this time, set "Game View Mode" to "Default".
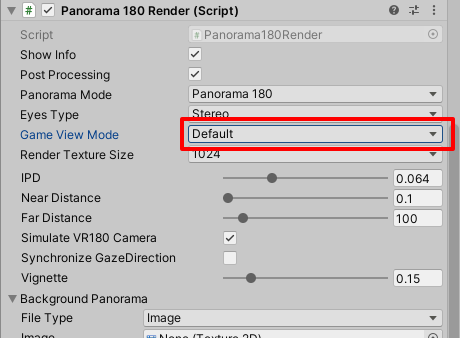
You can also specify "Game View Mode" as API, so you can leave it as "Panorama View".
This will create multiple cameras for the Panorama180 Render at runtime, but will not perform the panorama creation process.
By using the API, the panorama processing will be done at any given time.
Create a new GameObject and write a script to it.
Panorama180Renderのnamespace
To make it easier to access the Panorama180Render class, assign a namespace with the following specification.
using Panorama180Render = Panorama180Render.Panorama180Render;
Get the Panorama180Render component assigned to the camera
Get a reference to the Panorama180Render class from the main camera in Start().
The retrieved reference is stored in m_panorama180Render.
private Panorama180Render m_panorama180Render = null;
void Start () {
if (Camera.main != null) {
GameObject g = Camera.main.gameObject;
if (g != null) {
m_panorama180Render = g.GetComponent
();
}
}
}
Specify the initial value
Changes the default value of the Panorama180 Render.
If you have already specified a value in the UI of the Panorama180 Render assigned to the camera and do not need to change it,
you do not need to specify the following.
// Set GameViewMode (Default view).
m_panorama180Render.SetGameViewMode(Panorama180Render.GameViewMode.Default);
// Set IPD(Interpupillary distance. unit m).
m_panorama180Render.SetIPD(0.064f);
// Set texture size as Cubemap (512/1024/2048/4096/8192).
m_panorama180Render.SetRenderTextureSize(1024);
// Post Processing On / Off.
m_panorama180Render.SetPostProcessing(true);
// Output resolution at Panorama180.
m_panorama180Render.SetOutputTextureSizeType(Panorama180Render.OutputTextureSizeType._1024);
// Output resolution at Panorama360.
m_panorama180Render.SetOutputTextureSizeType360(Panorama180Render.OutputTextureSizeType360._2048);
SetGameViewMode(Panorama180Render.GameViewMode.Default)" specifies that the panorama image will not be drawn in front of the screen.
"SetIPD(0.064f)" specifies the IPD (unit: m).
"SetRenderTextureSize(1024)" specifies the resolution of a single surface as a cube map when creating a panorama.
If this value is too large, it will take a long time.
"SetPostProcessing(true)" will cause the panorama to reflect Post Processing if it exists (for built-in/URP).
"SetOutputTextureSizeType(Panorama180Render.OutputTextureSizeType._1024)" specifies the size of the output panorama image.
This is the resolution specification for Panorama 180-3D.
"SetOutputTextureSizeType360(Panorama180Render.OutputTextureSizeType360._2048)" specifies the resolution of the panoramic image in panorama 360-3D.
Start capture
Start capturing with "BeginCapture()".
// Begin capture.
m_panorama180Render.BeginCapture();
There is no "EndCapture()" specified here, as it starts capturing immediately after Play and keeps this state until the end.
When "EndCapture()" is called, the capture will stop there.
Get the RenderTexture
Call "GetRenderTexture()" between "BeginCapture()" and "EndCapture()" to get the color texture (RenderTexture) of the panorama.
This RenderTexture will be updated every frame, but the reference will not change.
If the capture has not been started, null is returned.
RenderTexture can be obtained by updating each frame using Update(), OnGUI(), etc. as shown below.
// Get RenderTexture while capturing.
if (m_panorama180Render.IsCapture()) {
// Get RenderTexture.
RenderTexture rc = m_panorama180Render.GetRenderTexture();
}
"IsCapture()" acquires a bool value to determine if the capture has started.
"GetRenderTexture()" to get the RenderTexture of the panorama.
The method of calling "GetRenderTexture()" between "BeginCapture()" and "EndCapture()" is more demanding because it constantly generates a panorama image.
In this section, the demo scene "Runtime/blocks_sync_RenderTexture" will be used as an example.
This is the process of using a coroutine to create and acquire a panoramic image once.
In this case, there is a load on a few frames during capture, but other than that, Panorama180 Render does not perform any processing, making it easy to handle in VR.
The aforementioned "BeginCapture()" and "EndCapture()" are not used.
The flow up to getting the reference to the Panorama180Render class in Start() is the same as above.
Specify the initial value
Changes the default value of the Panorama180 Render.
// Set GameViewMode (Default view).
m_panorama180Render.SetGameViewMode(Panorama180Render.GameViewMode.Default);
// Set IPD(Interpupillary distance. unit m).
m_panorama180Render.SetIPD(0.064f);
// Camera near,far distance.
m_panorama180Render.SetCameraNearFarDistance(0.1f, 10.0f);
// Set texture size as Cubemap (512/1024/2048/4096/8192).
// The performance was not good on Oculus Quest2
// if it was more than 1024 in SetRenderTextureSize() and 1024(Output 2048 x 1024) in SetOutputTextureSizeType().
m_panorama180Render.SetRenderTextureSize(1024);
// Post Processing On / Off.
m_panorama180Render.SetPostProcessing(true);
// Output resolution at Panorama180.
m_panorama180Render.SetOutputTextureSizeType(Panorama180Render.OutputTextureSizeType._1024);
// Set Panorama180.
m_panorama180Render.SetPanoramaMode(Panorama180Render.PanoramaMode.Panorama180);
// Set depth texture type.
m_panorama180Render.SetDepthTextureType(Panorama180Render.OutputDepthTextureType.DepthDefault);
// Set depth texture linear.
m_panorama180Render.SetDepthLinearType(Panorama180Render.OutputDepthLinearType.Linear);
The specification of the same parameters has been omitted.
"SetCameraNearFarDistance(0.1f, 10.0f)" specifies the distance to the near clip plane and the distance to the far clip plane (unit: m).
This is specified to adjust the shading of the Depth texture.
"SetPanoramaMode(Panorama180Render.PanoramaMode.Panorama180)" to capture in Panorama 180-3D.
"SetDepthTextureType(Panorama180Render.OutputDepthTextureType.DepthDefault)" also creates a depth texture for the panorama.
If you do not need a depth texture, specify "SetDepthTextureType(Panorama180Render.OutputDepthTextureType.None)".
"SetDepthLinearType(Panorama180Render.OutputDepthLinearType.Linear)" specifies the interpolation method for depth texture values.
Within Start(), the panorama capture process is not started.
Write a coroutine that performs a single capture
The capture process is performed by a coroutine.
IEnumerator GetPanoramaRenderTextureCo () {
if (m_panorama180Render == null) yield return null;
// Call coroutine.
var fc = m_panorama180Render.GetRenderTextureCo();
var curCoroutine = StartCoroutine(fc);
// Wait coroutine.
yield return curCoroutine;
// Get RenderTexture.
// The RenderTexture that can be obtained is temporary.
Panorama180Render.PanoramaTextureData rt = (Panorama180Render.PanoramaTextureData)fc.Current;
}
If m_panorama180Render is null, call "yield return null" to exit the coroutine.
Use "var fc = m_panorama180Render.GetRenderTextureCo();" to get the function of the coroutine,
and "var curCoroutine = StartCoroutine(fc);" to start the coroutine.
The "yield return curCoroutine;" waits for the coroutine to finish.
The coroutine creates a color texture and (if specified) a Depth texture for the panorama as a RenderTexture.
Once the panorama image has been created, the panorama creation process will stop.
This process may take several frames to complete.
Use "Panorama180Render.PanoramaTextureData rt = (Panorama180Render.PanoramaTextureData)fc.Current;" to get the resulting RenderTexture.
"rt.colorTexture" will be the color texture (RenderTextureFormat.ARGB32)。
"rt.depthTexture" will be the depth texture (RenderTextureFormat.ARGBFloat)。
Call Coroutine
In the case of this demo scene, the aforementioned coroutine is called when a button on the keyboard or gamepad is pressed.
StartCoroutine(GetPanoramaRenderTextureCo());
This process produces a panoramic RenderTexture with as little load as possible.
The following functions are provided for runtime.
Most of the features are the same as in the Editor, but file saving and other features are not available at runtime.
int GetVersion ();
Get the version of "Panorama180Render".
In the case of hexadecimal "0x1020", it becomes ver.1.0.2.
void SetRenderTextureSize (int texSize);
Specifies the texture size in one face of cube map.
Argument name | Details |
texSize | 512, 1024, 2048, 4096, 8192 |
int GetRenderTextureSize ();
Gets the texture size in one plane of cube map.
It can be 512, 1024, 2048, 4096 or 8192.
void SetOutputAlignType (AlignType type);
Specifies the type of arrangement of the panorama at the time of output.
Argument name | Details |
type |
Align type
AlignType.TopAndBottom ... up and down
AlignType.SideBySide ... left and right
|
AlignType GetOutputAlignType ();
Gets the type of arrangement of the panorama at the time of output.
AlignType.TopAndBottom is placed up and down, AlignType.SideBySide is placed on the left and right.
void SetOutputTextureSizeType (OutputTextureSizeType sType);
Specifies the output texture size type for Panorama 180-3D.
Argument name | Details |
sType |
Type of output texture size.
OutputTextureSizeType._512 ... 512*2 x 512 or 512 x 512*2
OutputTextureSizeType._1024 ... 1024*2 x 1024 or 1024 x 1024*2
OutputTextureSizeType._2048 ... 2048*2 x 2048 or 2048 x 2048*2
OutputTextureSizeType._4096 ... 4096*2 x 4096 or 4096 x 4096*2
OutputTextureSizeType._4K ... 3840 x 2160 (Side by side only)
OutputTextureSizeType._5_7K ... 5760 x 2880 (Side by side only)
OutputTextureSizeType._8K ... 7680 x 4320 (Side by side only)
OutputTextureSizeType._16K ... 15360 x 8640 (Side by side only)
OutputTextureSizeType._16384 ... 16384 x 8192 (Side by side only)
|
OutputTextureSizeType GetOutputTextureSizeType ();
Get the output texture size type for Panorama 180-3D.
This is the size of the texture when placing the two-eye panorama that will eventually be output.
OutputTextureSizeType._512, OutputTextureSizeType._1024, OutputTextureSizeType._2048, OutputTextureSizeType._4096,
OutputTextureSizeType._4K, OutputTextureSizeType._5_7K, OutputTextureSizeType._8K, OutputTextureSizeType._16K or OutputTextureSizeType._16384 is returned.
void SetPostProcessing (bool enableF);
Specifies Post Processing On/Off.
Argument name | Details |
enableF | true on, false off |
bool GetPostProcessing ();
Get Post Processing On/Off.
void SetDepthTextureType (OutputDepthTextureType type);
Specifies the type to output Depth.
Argument name | Details |
type |
Type when outputting Depth
OutputDepthTextureType.None ... Do not output
OutputDepthTextureType.DepthDefault ... Same size as panorama image
OutputDepthTextureType.DepthOneHalf ... 1/2 size
OutputDepthTextureType.DepthOneQuarter ... 1/4 size
|
OutputDepthTextureType GetDepthTextureType ();
Gets the type when outputting Depth.
OutputDepthTextureType.None does not output depth.
OutputDepthTextureType.DepthDefault outputs the same size as the panorama image.
OutputDepthTextureType.DepthOneHalf outputs with 1/2 size of panorama image.
OutputDepthTextureType.DepthOneQuarter outputs with 1/4 size of panorama image.
void SetDepthLinearType (OutputDepthLinearType type);
Specifies the type of interpolation at depth output.
Argument name | Details |
type |
Interpolation type when outputting depth
OutputDepthLinearType.Linear ... linear
OutputDepthLinearType.NonLinear ... non-linear
|
OutputDepthLinearType GetDepthLinearType ();
Gets the type of interpolation at depth output.
OutputDepthLinearType.Linear is linear.
OutputDepthLinearType.NonLinear is non-linear.
void SetCameraNearFarDistance (float nearP, float farP);
Specifies the distance of the camera near / far plane.
Argument name | Details |
nearP | Distance to near clip plane |
farP | Distance to far clip plane |
void SetSynchronizeGazeDirection (bool b);
This function was added in ver.1.1.0.
Specify whether XZ axis rotation is reflected in addition to Y axis rotation when panoramic image is generated.
Argument name | Details |
b | If true, the camera's XYZ rotation is reflected. If false, only the camera's Y rotation is reflected. |
bool IsSynchronizeGazeDirection ();
This function was added in ver.1.1.0.
If it is true, the XYZ rotation of the camera is reflected when generating panorama images.
If it is false, only the Y rotation of the camera is reflected.
void SetIPD (float t);
Specify the distance between the two eyes (IPD: interpupillary distance).
Argument name | Details |
t |
IPD (Unit : m)
|
float GetIPD ();
Get the distance between the two eyes (IPD: interpupillary distance).
void SetSimulateVR180Camera (bool b);
Specifies whether to process the panorama like a VR180 camera when using Panorama 180-3D.
Argument name | Details |
b |
If true, it will process the panorama like a VR180 camera.
In the case of false, the parallax when facing left or right is also interpolated as much as possible.
|
bool IsSimulateVR180Camera ();
When panorama 180-3D, returns true if the panorama is processed like a VR180 camera.
In the case of false, the parallax when facing left or right is also interpolated as much as possible.
void SetGameViewMode (GameViewMode vMode);
This function was added in ver.2.0.0.
Specify the "Game View Mode".
Argument name | Details |
vMode |
GameViewMode.Default ... default view
GameViewMode.PanoramaView ... panoramic view
|
GameViewMode GetGameViewMode ();
This function was added in ver.2.0.0.
Get the "Game View Mode" specification.
GameViewMode.Default/GameViewMode.PanoramaView will be returned.
void SetPanoramaMode (PanoramaMode pMode);
This function was added in ver.2.0.0.
Specify the "Panorama Mode" (Panorama 180-3D, Panorama 360-3D).
Argument name | Details |
vMode |
PanoramaMode.Panorama180 ... Panorama 180-3D
PanoramaMode.Panorama360 ... Panorama 360-3D
|
PanoramaMode GetPanoramaMode ();
This function was added in ver.2.0.0.
Get the "Panorama Mode" (Panorama 180-3D, Panorama 360-3D).
PanoramaMode.Panorama180/PanoramaMode.Panorama360 will be returned.
void SetOutputTextureSizeType360 (OutputTextureSizeType360 sType);
This function was added in ver.2.0.0.
Specifies the output texture size type for Panorama 360-3D.
Argument name | Details |
sType |
Output texture size type.
OutputTextureSizeType360._512 ... 512 x 512
OutputTextureSizeType360._1024 ... 1024 x 1024
OutputTextureSizeType360._2048 ... 2048 x 2048
OutputTextureSizeType360._3072 ... 3072 x 3072
OutputTextureSizeType360._4096 ... 4096 x 4096
OutputTextureSizeType360._4K ... 3840 x 3840
OutputTextureSizeType360._5_7K ... 5760 × 5760
OutputTextureSizeType360._8K ... 7680 x 7680
OutputTextureSizeType360._16K ... 15360 x 15360
OutputTextureSizeType360._16384 ... 16384 x 16384
|
OutputTextureSizeType360 GetOutputTextureSizeType360 ();
This function was added in ver.2.0.0.
Get the output texture size type for Panorama 360-3D.
This is the size of the texture when placing the two-eye panorama that will eventually be output.
OutputTextureSizeType360._512, OutputTextureSizeType360._1024, OutputTextureSizeType360._2048,
OutputTextureSizeType360._3072, OutputTextureSizeType360._4096, OutputTextureSizeType360._4K,
OutputTextureSizeType360._5_7K, OutputTextureSizeType360._8K, OutputTextureSizeType360._16K or
OutputTextureSizeType360._16384 is returned
void SetEyesType (EyesType eType);
This function was added in ver.2.0.1.
Specifies monocular or stereo.
Argument name | Details |
eType |
Monocular/stereo types
EyesType.Stereo ... Stereo (Default)
EyesType.Mono ... Monocular
|
EyesType GetEyesType ();
This function was added in ver.2.0.1.
Get monocular or stereo.
EyesType.Stereo, EyesType.Mono is returned.
void BeginCapture ();
This function was added in ver.2.0.0.
Start the panorama capture process.
void EndCapture ();
This function was added in ver.2.0.0.
End the panorama capture process.
bool IsCapture ();
This function was added in ver.2.0.0.
Returns true if panorama processing is in progress (between BeginCapture() and EndCapture()).
RenderTexture GetRenderTexture ();
This function was added in ver.2.0.0.
When panorama processing is in progress (between BeginCapture() and EndCapture()), this function gets the target RenderTexture.
IEnumerator GetRenderTextureCo (bool endCaptureF = true);
This function was added in ver.2.0.0.
Get the panoramic image. This is called from a coroutine.
Argument name | Details |
endCaptureF |
If true, the camera for capture will be hidden when the capture is completed so that the capture process will not be performed.
If false, the capture process will continue as is.
|
The resulting panoramic image is retrieved as "Panorama180Render.PanoramaTextureData rt = (Panorama180Render.PanoramaTextureData)fc.Current;" as the return value of the coroutine.
"rt.colorTexture" will be the color texture(RenderTextureFormat.ARGB32).
"rt.depthTexture" will be the depth texture(RenderTextureFormat.ARGBFloat).
See "
Usage : Use coroutine" for instructions.